Golang, also known as Go, has quickly become a favorite among developers for its speed, simplicity, and efficiency. Created by Google, this statically typed, compiled language is ideal for large-scale projects, offering excellent concurrency support and a clean, straightforward syntax.
Whether you’re an experienced developer or just starting, working on practical projects is the best way to enhance your skills. In this article, we will explain the 35+ Exciting Golang Project Ideas to Boost Your Skills, which presents a variety of projects suited for different skill levels.
These project ideas range from simple applications to complex systems, ensuring you find something that matches your expertise and learning goals.
Engaging with these projects will deepen your understanding of Golang and provide valuable hands-on experience. Each project is designed to help you grasp core concepts, utilize Golang’s unique features, and become a more proficient developer.
Whether you aim to build a basic web server, develop a sophisticated distributed system, or contribute to open-source projects, these Golang project ideas will give you the practical experience needed to boost your skills and advance your career.
What Is Golang?
Golang, or Go, is an open-source programming language developed by Google and released in 2009. Created by Robert Griesemer, Rob Pike, and Ken Thompson, it is known for its simplicity, efficiency, and performance. Golang is popular for developing a wide range of applications, from small projects to large-scale distributed systems.
Key Features of Golang:
- Concurrency: Golang supports concurrent programming, allowing programs to perform multiple tasks simultaneously with goroutines and channels, which are simple yet powerful tools for managing concurrency.
- Fast Compilation: Golang is a statically typed, compiled language, meaning it compiles directly into machine code. This results in fast execution and efficient resource use.
- Simple Syntax: olang’s syntax is designed for simplicity, making it easy to learn and use. This reduces code complexity and improves readability.
- Rich Standard Library: Golang includes a comprehensive standard library with many built-in functions and packages, facilitating common tasks without needing external libraries.
- Garbage Collection: Golang has an efficient garbage collector that automatically manages memory allocation and deallocation, preventing memory leaks and enhancing program stability.
- Strong Typing: Golang enforces strong typing, which catches errors at compile time rather than runtime, improving code reliability and reducing bugs.
- Cross-Platform Support: Golang supports cross-platform development, allowing code to run on different operating systems, including Windows, macOS, and Linux, without modification.
Use Cases of Golang:
- Web Development: Building robust and scalable web servers and APIs.
- Cloud Services: Developing cloud-native applications and microservices.
- Networking: Creating efficient networking tools and services.
- DevOps Tools: Developing powerful tools for system administration and automation.
- Data Processing: Efficiently handling large-scale data processing tasks.
Golang’s combination of performance, simplicity, and powerful features makes it an excellent choice for various applications. Its growing popularity highlights its effectiveness and versatility.
Also Read: 99+ Latest ATL Project Ideas for Students Updated 2024 |
35+ Exciting Golang Project Ideas to Boost Your Skills In 2024
Here are 35+ exciting Golang project ideas to boost your skills in 2024. These projects range from beginner to advanced levels and cover various aspects of Golang, including web development, networking, concurrency, and more.
Beginner Projects
- Simple Web Server
- Description: Build a basic web server that can handle HTTP requests and serve static files.
- What You’ll Learn: HTTP server basics, routing, handling requests and responses.
- What You Need: Golang, basic knowledge of HTTP protocols.
- Key Features: Serve static files, basic routing, request handling.
- To-Do List Application
- Description: Create a CLI or web-based application to manage tasks with features for adding, viewing, and deleting tasks.
- What You’ll Learn: Data storage, CRUD operations, basic UI/CLI development.
- What You Need: Golang, knowledge of file handling or simple databases.
- Key Features: Task management, persistent storage, task editing.
- Basic Calculator
- Description: Develop a calculator that performs fundamental arithmetic operations like addition, subtraction, multiplication, and division.
- What You’ll Learn: Basic arithmetic operations, user input handling.
- What You Need: Golang, basic understanding of arithmetic.
- Key Features: Arithmetic operations, user input processing.
- URL Shortener
- Description: Build a service that shortens long URLs and redirects users to the original link.
- What You’ll Learn: URL handling, data storage, redirection.
- What You Need: Golang, basic understanding of URL structures.
- Key Features: URL shortening, redirection, unique link generation.
- Weather App
- Description: Create an app that fetches and displays weather information from a public API.
- What You’ll Learn: API integration, JSON parsing, data display.
- What You Need: Golang, knowledge of APIs, and JSON.
- Key Features: Fetch weather data, display current conditions, handle API responses.
- Chatbot
- Description: Implement a simple chatbot that can respond to predefined questions or engage in basic conversation.
- What You’ll Learn: Basic AI principles, natural language processing, interaction design.
- What You Need: Golang, basic understanding of chatbot frameworks.
- Key Features: Predefined responses, simple conversational flow.
- Number Guessing Game
- Description: Develop a command-line game where the player guesses a randomly generated number within a range.
- What You’ll Learn: Random number generation, user input handling, game logic.
- What You Need: Golang, basic game development concepts.
- Key Features: Number generation, user guesses, win/loss conditions.
- Expense Tracker
- Description: Build an application to track and categorize personal expenses, generating summaries or reports.
- What You’ll Learn: Data categorization, report generation, persistent storage.
- What You Need: Golang, knowledge of file handling or databases.
- Key Features: Expense entry, categorization, reporting.
- Simple File Backup Tool
- Description: Create a tool to back up files from one directory to another, including basic error handling.
- What You’ll Learn: File operations, error handling, directory management.
- What You Need: Golang, basic file system knowledge.
- Key Features: File copying, error handling, directory management.
- Markdown to HTML Converter
- Description: Develop a tool that converts Markdown files into HTML format.
- What You’ll Learn: Markdown parsing, HTML generation, file handling.
- What You Need: Golang, knowledge of Markdown syntax.
- Key Features: Markdown parsing, HTML output, file conversion.
Intermediate Projects
- RESTful API
- Description: Build a RESTful API for a simple service like a blog or task manager, supporting CRUD operations.
- What You’ll Learn: API design, HTTP methods, JSON handling.
- What You Need: Golang, understanding of REST principles.
- Key Features: CRUD operations, API endpoints, JSON responses.
- Chat Application
- Description: Develop a real-time chat application using WebSockets to enable live communication between users.
- What You’ll Learn: WebSocket protocol, real-time communication, server-client interaction.
- What You Need: Golang, knowledge of WebSocket basics.
- Key Features: Real-time messaging, user sessions, WebSocket connections.
- File Server
- Description: Create a server that allows users to upload, download, and list files.
- What You’ll Learn: File management, HTTP handling, server-side operations.
- What You Need: Golang, knowledge of HTTP file handling.
- Key Features: File upload/download, file listing, server-side file management.
- CLI Todo List Manager
- Description: Build a command-line tool to manage a to-do list with features like adding, deleting, and listing tasks.
- What You’ll Learn: CLI development, task management, data persistence.
- What You Need: Golang, familiarity with CLI tools.
- Key Features: Task management, CLI commands, persistent storage.
- URL Monitoring Service
- Description: Develop a service that periodically checks the status of URLs and alerts users if they are down.
- What You’ll Learn: URL monitoring, alerting mechanisms, scheduling tasks.
- What You Need: Golang, knowledge of HTTP requests and scheduling.
- Key Features: URL status checks, alert notifications, periodic checks.
- Simple Blog Engine
- Description: Create a blog engine with features for creating, editing, and deleting posts, along with user authentication.
- What You’ll Learn: Content management, user authentication, CRUD operations.
- What You Need: Golang, understanding of web development concepts.
- Key Features: Post management, user authentication, content editing.
- Library Management System
- Description: Build a system to manage books, users, and borrowing records for a library.
- What You’ll Learn: Database design, record management, user management.
- What You Need: Golang, knowledge of databases.
- Key Features: Book cataloging, user management, borrowing records.
- Image Resizer
- Description: Develop a tool that resizes images to specified dimensions or formats.
- What You’ll Learn: Image processing, file handling, resizing algorithms.
- What You Need: Golang, understanding of image processing libraries.
- Key Features: Image resizing, format conversion, file management.
- Tic-Tac-Toe Game
- Description: Implement a command-line version of Tic-Tac-Toe with a basic AI opponent.
- What You’ll Learn: Game logic, AI development, user interface design.
- What You Need: Golang, basic game development concepts.
- Key Features: Game board, player input, basic AI.
- Notes Application
- Description: Create a note-taking application with features for categorizing and searching notes.
- What You’ll Learn: Data storage, search functionality, user interface design.
- What You Need: Golang, understanding of file handling or databases.
- Key Features: Note management, categorization, search functionality.
Advanced Projects
- Distributed System
- Description: Build a distributed system to demonstrate data replication, partitioning, and fault tolerance.
- What You’ll Learn: Distributed computing concepts, data consistency, fault tolerance.
- What You Need: Golang, knowledge of distributed systems principles.
- Key Features: Data replication, partitioning, fault tolerance.
- Blockchain Implementation
- Description: Develop a basic blockchain with features like block creation, transaction handling, and consensus algorithms.
- What You’ll Learn: Blockchain principles, consensus algorithms, transaction processing.
- What You Need: Golang, understanding of blockchain technology.
- Key Features: Block creation, transaction handling, consensus.
- Microservices Architecture
- Description: Create a set of microservices that communicate and perform various tasks, like user management and data processing.
- What You’ll Learn: Microservices design, inter-service communication, scalability.
- What You Need: Golang, knowledge of microservices architecture.
- Key Features: Service communication, scalability, independent deployments.
- Machine Learning Model Deployment
- Description: Deploy a machine learning model as a service that makes predictions based on input data.
- What You’ll Learn: Model deployment, API integration, machine learning basics.
- What You Need: Golang, understanding of machine learning models.
- Key Features: Model prediction, API integration, data handling.
- API Rate Limiter
- Description: Build a rate-limiting service to control the number of API requests a user can make within a specified timeframe.
- What You’ll Learn: Rate limiting algorithms, API management, request tracking.
- What You Need: Golang, knowledge of API design and rate limiting.
- Key Features: Request tracking, rate limiting, API protection.
- Custom Web Framework
- Description: Develop a simple web framework to handle routing, middleware, and request processing.
- What You’ll Learn: Web framework design, routing, middleware implementation.
- What You Need: Golang, understanding of web server concepts.
- Key Features: Routing, middleware support, request processing.
- Real-Time Data Streaming Application
- Description: Create an application that processes and displays real-time data streams, such as stock prices or social media feeds.
- What You’ll Learn: Real-time data processing, streaming technologies, data visualization.
- What You Need: Golang, knowledge of data streaming and processing.
- Key Features: Real-time updates, data processing, visualization.
- Load Balancer
- Description: Implement a load balancer that distributes incoming network traffic across multiple servers.
- What You’ll Learn: Load balancing techniques, traffic distribution, server management.
- What You Need: Golang, understanding of network traffic management.
- Key Features: Traffic distribution, load balancing, server health checks.
- Service Discovery System
- Description: Build a system for services to register and discover each other dynamically.
- What You’ll Learn: Service registration, discovery mechanisms, dynamic configuration.
- What You Need: Golang, knowledge of service discovery concepts.
- Key Features: Service registration, dynamic discovery, health checks.
- Log Aggregator
- Description: Develop a tool to collect and analyze logs from various sources, providing insights and visualizations.
- What You’ll Learn: Log collection, aggregation, data analysis.
- What You Need: Golang, knowledge of log management and analysis.
- Key Features: Log collection, aggregation, visualization.
- Event-Driven Architecture
- Description: Create an application that uses an event-driven architecture to handle and process events asynchronously.
- What You’ll Learn: Event-driven design, asynchronous processing, message handling.
- What You Need: Golang, understanding of event-driven principles.
- Key Features: Event handling, asynchronous processing, messaging.
- Video Streaming Service
- Description: Build a service for video uploads, storage, and streaming to users.
- What You’ll Learn: Video processing, streaming protocols, media storage.
- What You Need: Golang, knowledge of video streaming technologies.
- Key Features: Video uploads, streaming, storage management.
- Secure File Sharing Platform
- Description: Develop a platform for securely sharing files with encryption and access control.
- What You’ll Learn: File encryption, secure sharing, access control mechanisms.
- What You Need: Golang, understanding of encryption and security practices.
- Key Features: Encryption, secure access, file sharing.
- Custom ORM (Object-Relational Mapping) Library
- Description: Create a library that simplifies database interactions by mapping database tables to Go structs.
- What You’ll Learn: ORM concepts, database interactions, mapping techniques.
- What You Need: Golang, knowledge of databases, and ORM principles.
- Key Features: Database mapping, CRUD operations, query building.
- AI-Powered Recommendation System
- Description: Build a recommendation system that uses machine learning algorithms to suggest products or content based on user preferences.
- What You’ll Learn: Recommendation algorithms, machine learning, data analysis.
- What You Need: Golang, understanding of machine learning models.
- Key Features: Recommendation algorithms, user preference analysis, and content suggestions.
- Distributed Cache System
- Description: Develop a distributed caching system to improve data retrieval performance across multiple servers.
- What You’ll Learn: Caching techniques, distributed systems, performance optimization.
- What You Need: Golang, knowledge of caching strategies and distributed systems.
- Key Features: Data caching, distribution, performance enhancement.
- Serverless Function Framework
- Description: Create a framework for deploying and managing serverless functions, similar to AWS Lambda.
- What You’ll Learn: Serverless architecture, function deployment, management.
- What You Need: Golang, understanding of serverless computing.
- Key Features: Function deployment, management, scalability.
These projects will help you deepen your understanding of Golang, tackle real-world challenges, and build a diverse portfolio.
Also Read: 29+ Operating System Project Ideas for Students (2024) |
Tips for Choosing the Right Project
Selecting the right Golang project is crucial for enhancing your skills and achieving your learning goals. Here are some tips to help you make the best choice:
- Assess Your Skill Level
- Beginner: If you’re new to Golang, start with simple projects like a basic web server, to-do list application, or number guessing game. These will help you get comfortable with the syntax and fundamental concepts.
- Intermediate: With some experience, choose projects like developing a RESTful API, a chat application, or a simple blog engine to enhance your web development and API integration skills.
- Advanced: For seasoned developers, tackle complex projects like building a distributed system, implementing a blockchain, or creating a microservices architecture. These will challenge you and deepen your understanding of advanced topics.
- Identify Your Interests
- Choose projects that align with your interests. If you enjoy web development, consider building web applications or APIs. If you’re fascinated by data, explore projects related to data processing or machine learning.
- Set Clear Goals
- Define what you want to achieve with your project. Are you looking to learn a new concept, solve a specific problem, or build something for your portfolio? Clear goals will help you stay focused and motivated.
- Consider Practicality and Usefulness
- Pick projects with practical applications or that can be useful to others. For example, a URL shortener or an expense tracker can be applied in real-life scenarios, adding value to your work.
- Evaluate Complexity and Time Commitment
- Be realistic about the complexity of the project and the time you can commit. Starting with smaller projects and gradually moving to more complex ones can help you build confidence and avoid frustration.
- Leverage Existing Resources
- Look for projects that have available tutorials, documentation, or community support. These resources can provide guidance and help you overcome challenges more efficiently.
- Focus on Key Learning Outcomes
- Choose projects that will help you learn or improve specific skills. For example, if you want to master concurrency in Golang, a project like a real-time data streaming application can be very beneficial.
- Balance Between Fun and Challenge
- Select a project that you find enjoyable but also challenging enough to push your boundaries. This balance will keep you engaged and promote continuous learning.
- Consider Collaboration Opportunities
- Some projects can be more fun and educational when done collaboratively. Look for opportunities to work with others, whether through open-source contributions, coding bootcamps, or project-based learning groups.
- Review and Iterate
- After completing a project, take the time to review what you’ve learned and identify areas for improvement. Use this reflection to choose your next project with a better understanding of your strengths and areas for growth.
By following these tips, you can select a Golang project that enhances your skills, keeps you motivated, and aligns with your learning objectives.
Wrap Up
Choosing the right Golang project is crucial for your development as a programmer. Start by assessing your skill level and identifying your interests to ensure the project is both engaging and educational. Set clear goals and select practical projects that add real value. Be realistic about the complexity and time required, and make use of existing resources for support.
Focus on specific learning outcomes, balance enjoyment with challenge, and explore collaboration opportunities for a richer experience. Finally, review your work and iterate for continuous improvement. By following these guidelines, you can choose projects that effectively enhance your Golang skills while keeping you motivated and aligned with your learning objectives.
FAQs
Are there benefits to collaborating on Golang projects?
Yes, collaborating with others can enhance your learning experience, provide different perspectives, and help you tackle larger, more complex projects.
What should I consider when setting project goals?
Define what you want to learn or achieve, such as mastering a new concept, solving a particular problem, or creating a portfolio piece. Clear goals help maintain focus and motivation throughout the project.
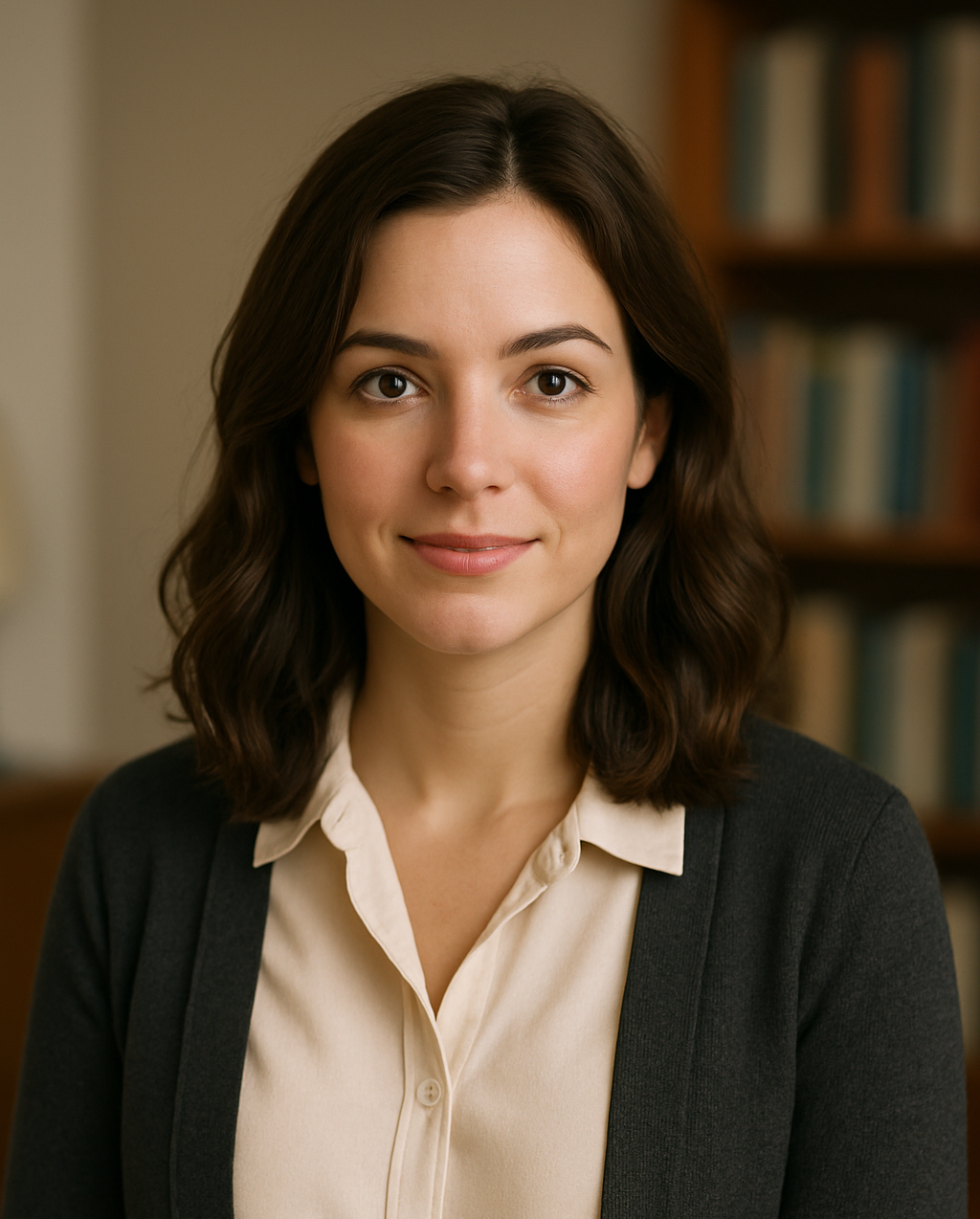
I’m Isla Campbell, a creative and passionate professional with over 8 years of experience in education and project-based learning. I enjoy coming up with smart, helpful project ideas that inspire students and support teachers. I’m skilled at doing research, finding what works best, and turning ideas into successful learning experiences. I also love working with others, staying organized, and making sure every project is done well and on time. Let’s team up to turn great ideas into real results!